二、Stream API:filter、distinct、skip、limit
被操作数据
List<Integer> list = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10,6,5,4,3,2,1);
1、filter 过滤
范例:
//filter 过滤
List<Integer> filterResult = list.stream().filter(i -> i % 2 == 0).collect(toList());
filterResult.stream().forEach(System.out::println);
2、distinct 去重
范例:
//distinct 去重
List<Integer> distinctResult = list.stream().distinct().collect(toList());
distinctResult.stream().forEach(System.out::println);
3、skip 截断
范例:
//skip 截断(跳过前面几个,超过长度,直接返回空)
List<Integer> skipResult = list.stream().skip(5).collect(toList());
skipResult.stream().forEach(System.out::println);
4、limit 查询几条
范例:
//limit 查询几条
List<Integer> limitResult = list.stream().limit(3).collect(toList());
limitResult.stream().forEach(System.out::println);
二、Stream API:Map
1、map 数据处理
被操作数据
List<Integer> list = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10,6,5,4,3,2,1);
List<Dish> menu = Arrays.asList(
new Dish("pork", false, 800, Dish.Type.MEAT),
new Dish("beef", false, 700, Dish.Type.MEAT),
new Dish("chicken", false, 400, Dish.Type.MEAT),
new Dish("french fries", true, 530, Dish.Type.OTHER),
new Dish("rice", true, 350, Dish.Type.OTHER),
new Dish("season fruit", true, 120, Dish.Type.OTHER),
new Dish("pizza", true, 550, Dish.Type.OTHER),
new Dish("prawns", false, 300, Dish.Type.FISH),
new Dish("salmon", false, 450, Dish.Type.FISH));
String[] arrays = {"Hello","world"};
范例1:
//1、map
List<Integer> mapResult = list.stream().map(i -> i * 2).collect(toList());
mapResult.stream().forEach(System.out::println);
范例2:
//2、map
menu.stream().map(Dish::getName).forEach(System.out::println);
范例3:
//3、flatMap 扁平化
//拆分成String[]: {h,e,l,l,o},{w,o,r,l,d}
Stream<String[]> splitStream = Arrays.stream(arrays).map(s -> s.split(""));
//flatMap扁平化,将{h,e,l,l,o},{w,o,r,l,d}每个数组转成Stream<String>
Stream<String> stringStream = splitStream.flatMap(Arrays::stream);
stringStream.distinct().forEach(System.out::println);
打印结果:
H
e
l
o
w
r
d
三、Stream API:Match
1、Match匹配
范例:
1.1、allMatch 全部满足条件
Stream<Integer> list = Arrays.stream(new Integer[]{1, 2, 3, 4, 5, 6, 7, 8, 9, 10});
//1、allMatch 全部满足条件
boolean booleanAnyMath = list.allMatch(i -> i > 0);
System.out.println(booleanAnyMath);
1.2、anyMatch 任意一个满足条件
//2、anyMatch 任意一个满足条件
list = Arrays.stream(new Integer[]{1, 2, 3, 4, 5, 6, 7, 8, 9, 10});
boolean anyMatch = list.anyMatch(i -> i > 9);
System.out.println(anyMatch);
1.3、noneMatch 没有一个满足
//3、noneMatch 没有一个满足
list = Arrays.stream(new Integer[]{1, 2, 3, 4, 5, 6, 7, 8, 9, 10});
boolean noneMatch = list.noneMatch(i -> i == 11);
System.out.println(noneMatch);
四、Stream API:find
2、find查找
范例:
2.1、findFirst 查找第一个
//1、findFirst 查找第一个
Optional<Integer> firstOptional = stream.filter(i -> i % 2 == 0).findFirst();
System.out.println(firstOptional);
````
**2.2、findAny 查找任意一个**
stream = Arrays.stream(new Integer[]{1, 2, 3, 4, 5, 6, 7, 8, 9, 10});
Optional<Integer> anyOptional = stream.filter(i -> i % 2 == 0).findAny();
System.out.println(anyOptional.get());
**2.3、没找到时,使用get()会直接抛出异常提示信息,会提示错误:No value present**
```java
stream = Arrays.stream(new Integer[]{1, 2, 3, 4, 5, 6, 7, 8, 9, 10});
Optional<Integer> noNumOptional = stream.filter(i -> i == 11).findAny();
System.out.println(noNumOptional.get());
抛出异常提示:
//抛出异常提示:No value present
````
**2.4、没找到时,Optional输出:Optional.empty,不抛异常**
System.out.println(noNumOptional);
*输出结果:*
Optional.empty
**3、orElse 如果没查找返回给定的值**
范例: **需求:查询数组中是否包含指定值,没有返回默认值。**
***3.1、原始写法***
先写个方法,然后调用
/*
* 原始写法,查询数组中是否包含指定值,没有返回默认值
* @param values
* @param defaultValue
* @param predicate
* @return
*/
public static int findDifineValue(Integer[] values, int defaultValue, Predicate<Integer> predicate){
for(int i : values){
if(predicate.test(i)){
return i;
}
}
return defaultValue;
}
调用原始写法:
int findValueResult = findDifineValue(new Integer[]{1, 2, 3, 4, 5, 6, 7, 8, 9, 10}, -1, i -> i == 9);
System.out.println(findValueResult);
***3.2、orElse写法***
// orElse写法 如果没查找返回给定的值
stream = Arrays.stream(new Integer[]{1, 2, 3, 4, 5, 6, 7, 8, 9, 10});
Optional<Integer> noNumOptional = stream.filter(i -> i == 11).findAny();
System.out.println(noNumOptional.orElse(-1));
比原始写法简写很多。
----------
**4、isPresent 判断是否存在,直接返回boolean值**
stream = Arrays.stream(new Integer[]{1, 2, 3, 4, 5, 6, 7, 8, 9, 10});
boolean isPresent = stream.filter(i->i==4).findAny().isPresent();
System.out.println(isPresent);
**5、存在就打印出来,没有则不打印**
stream = Arrays.stream(new Integer[]{1, 2, 3, 4, 5, 6, 7, 8, 9, 10});
stream.filter(i->i==6).findAny().ifPresent(System.out::println);
** 6、还可以进行二次过滤**
stream = Arrays.stream(new Integer[]{1, 2, 3, 4, 5, 6, 7, 8, 9, 10});
//先Stream的filter过滤,然后得到Optional,再用Optional的filter过滤
stream.filter(i->i>8).findAny().filter(i->i<10).ifPresent(System.out::println);
## 五、Stream API:reduce:聚合作用,根据reduce传入的Function条件进行聚合
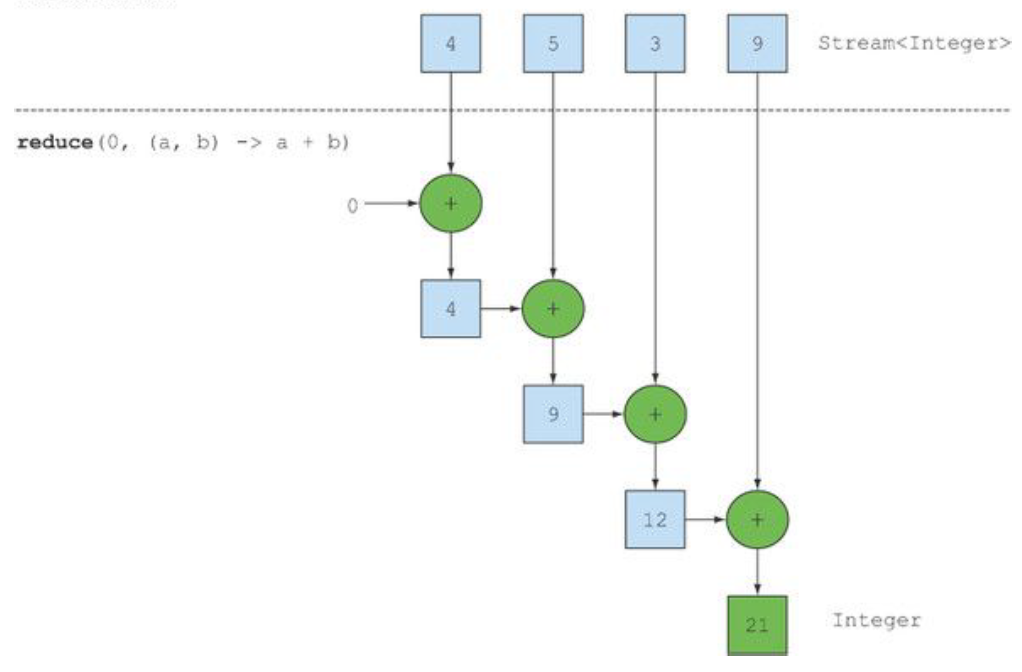
范例:
package com.example.study.java8.streams;
import java.util.Arrays;
import java.util.List;
/**
- Stream reduce:聚合作用,根据reduce传入的Function条件进行聚合
- 用法:只要reduce里面参数满足funcion就可以
*/
public class StreamReduce {
public static void main(String[] args) {
//用法:只要reduce的参数满足Function就可以
//reduce(0, (i, j) -> i + j)
List<Integer> list = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10,6,5,4,3,2,1);
Integer reduceResult = list.stream().reduce(0, (i, j) -> i + j);
System.out.println(reduceResult);
System.out.println("===================================");
//reduce((i,j)->i+j)
list = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10,6,5,4,3,2,1);
list.stream().reduce((i,j)->i+j).ifPresent(System.out::println);
System.out.println("===================================");
//reduce(Math::max)
list = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10,6,5,4,3,2,1);
list.stream().reduce(Math::max).ifPresent(System.out::println);
System.out.println("===================================");
//reduce(Integer::sum)
list = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10,6,5,4,3,2,1);
list.stream().reduce(Integer::sum).ifPresent(System.out::println);
}
}
评论 (0)